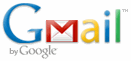
Here’s the list of Gmail IMAP protocol extensions implemented in Mail.dll:
- Extension of the LIST command: XLIST
- Extension of the SEARCH command: X-GM-RAW
- Access to the Gmail unique message ID: X-GM-MSGID
- Access to the Gmail thread ID: X-GM-THRID
- Access to Gmail labels: X-GM-LABELS
- OAuth and OAuth 2.0: XOAUTH, XOAUTH2
You can read on how to use Mail.dll with Gmail in the following articles:
OAuth 2.0
OAuth 1.0
Posted in Mail.dll | Tags: Gmail, IMAP, IMAP component, OAuth, OAuth 2.0, X-GM-LABELS, X-GM-MSGID, X-GM-RAW, X-GM-THRID, XLIST, XOAuth, XOAUTH2
Gmail treats labels as folders for the purposes of IMAP.
As such, labels can be modified using the standard IMAP commands, CreateFolder, RenameFolder, and DeleteFolder, that act on folders.
System labels, which are labels created by Gmail, are reserved and prefixed by “[Gmail]” or “[GoogleMail]” in the list of labels.
Use the XLIST command to get the entire list of labels for a mailbox.
The labels for a given message may be retrieved by using the X-GM-LABELS attribute with the FETCH command.
It is also possible to use the X-GM-LABELS attribute to return the UIDs for all messages with a given label, using the SEARCH command.
// C# version
using (Imap imap = new Imap())
{
imap.ConnectSSL("imap.gmail.com");
imap.UseBestLogin("pat@gmail.com", "password");
// Select 'All Mail' folder
CommonFolders common = new CommonFolders(client.GetFolders());
client.Select(common.AllMail);
List<long> uids = imap.Search().Where(
Expression.GmailLabel("MyLabel"));
foreach (MessageInfo info in imap.GetMessageInfoByUID(uids))
{
Console.WriteLine("{0} - {1}",
info.Envelope.GmailThreadId,
info.Envelope.Subject);
}
imap.Close();
}
' VB.NET version
Using imap As New Imap()
imap.ConnectSSL("imap.gmail.com")
imap.UseBestLogin("pat@gmail.com", "password")
' Select 'All Mail' folder
Dim common As New CommonFolders(client.GetFolders())
client.Select(common.AllMail)
Dim uids As List(Of Long) = imap.Search().Where(Expression.GmailLabel("MyLabel"))
For Each info As MessageInfo In imap.GetMessageInfoByUID(uids)
Console.WriteLine("{0} - {1}", _
info.Envelope.GmailThreadId, _
info.Envelope.Subject)
Next
imap.Close()
End Using
You can learn more about this Gmail IMAP extension here:
http://code.google.com/apis/gmail/imap/#x-gm-labels
Posted in Mail.dll | Tags: C#, Gmail, IMAP, IMAP component, VB.NET, X-GM-LABELS
If you want to use system label, such as \Starred or \Important please read label message with system label.
Gmail treats labels as folders for the purposes of IMAP.
As such, labels can be modified using the standard IMAP commands, CreateFolder, RenameFolder, and DeleteFolder, that act on folders.
System labels, which are labels created by Gmail, are reserved and prefixed by “[Gmail]” or “[GoogleMail]” in the list of labels.
Use the XLIST command to get the entire list of labels for a mailbox.
The labels for a given message may be retrieved by using the X-GM-LABELS attribute with the FETCH command.
Labels may be added to a message using the STORE command in conjunction with the X-GM-LABELS attribute.
// C# version
using (Imap imap = new Imap())
{
imap.ConnectSSL("imap.gmail.com");
imap.UseBestLogin("pat@gmail.com", "password");
imap.SelectInbox();
long uid = imap.GetAll().Last();
imap.GmailLabelMessageByUID(uid, "MyLabel");
List<string> labels = imap.GmailGetLabelsByUID(uid);
labels.ForEach(Console.WriteLine);
imap.Close();
}
' VB.NET version
Using imap As New Imap()
imap.ConnectSSL("imap.gmail.com")
imap.UseBestLogin("pat@gmail.com", "password")
imap.SelectInbox()
Dim uid As Long = imap.GetAll().Last()
imap.GmailLabelMessageByUID(uid, "MyLabel")
Dim labels As List(Of String) = imap.GmailGetLabelsByUID(uid)
labels.ForEach(Console.WriteLine)
imap.Close()
End Using
You can learn more about this Gmail IMAP extension here:
http://code.google.com/apis/gmail/imap/#x-gm-labels
Posted in Mail.dll | Tags: C#, Gmail, IMAP, IMAP component, VB.NET, X-GM-LABELS
Gmail treats labels as folders for the purposes of IMAP.
As such, labels can be modified using the standard IMAP commands, CreateFolder, RenameFolder, and DeleteFolder, that act on folders.
System labels, which are labels created by Gmail, are reserved and prefixed by “[Gmail]” or “[GoogleMail]” in the list of labels.
Use the XLIST command to get the entire list of labels for a mailbox.
The labels for a given message may be retrieved by using the X-GM-LABELS attribute with the FETCH command.
For list of messages
// C# version
using (Imap imap = new Imap())
{
imap.ConnectSSL("imap.gmail.com");
imap.UseBestLogin("pat@gmail.com", "password");
imap.SelectInbox();
List<long> uids = imap.GetAll();
List<messageInfo> infos = imap.GetMessageInfoByUID(uids);
foreach (MessageInfo info in infos)
{
List<string> labels = info.GmailLabels.Labels;
Console.WriteLine("[{0}] {1}",
string.Join(" ", labels.ToArray()),
info.Envelope.Subject);
}
imap.Close();
}
' VB.NET version
Using imap As New Imap()
imap.ConnectSSL("imap.gmail.com")
imap.UseBestLogin("pat@gmail.com", "password")
imap.SelectInbox()
Dim uids As List(Of Long) = imap.GetAll()
Dim infos As List(Of MessageInfo) = imap.GetMessageInfoByUID(uids)
For Each info As MessageInfo In infos
Dim labels As List(Of String) = info.GmailLabels.Labels
Console.WriteLine("[{0}] {1}", _
String.Join(" ", labels.ToArray()), _
info.Envelope.Subject)
Next
imap.Close()
End Using
For single message:
// C# version
using (Imap imap = new Imap())
{
imap.ConnectSSL("imap.gmail.com");
imap.Login("pat@gmail.com", "password");
imap.SelectInbox();
long uid = imap.GetAll().Last();
List<string> labels = imap.GmailGetLabelsByUID(uid);
labels.ForEach(Console.WriteLine);
imap.Close();
}
' VB.NET version
Using imap As New Imap()
imap.ConnectSSL("imap.gmail.com")
imap.Login("pat@gmail.com", "password")
imap.SelectInbox()
Dim uid As Long = imap.GetAll().Last()
Dim labels As List(Of String) = imap.GmailGetLabelsByUID(uid)
labels.ForEach(Console.WriteLine)
imap.Close()
End Using
You can learn more about this Gmail IMAP extension here:
http://code.google.com/apis/gmail/imap/#x-gm-labels
Posted in Mail.dll | Tags: C#, Gmail, IMAP, IMAP component, VB.NET, X-GM-LABELS
To provide access to the full Gmail search syntax, Gmail provides the X-GM-RAW search attribute.
Arguments passed along with the X-GM-RAW attribute when executing the SEARCH command will be interpreted in the same manner as in the Gmail web interface.
// C# version
using (Imap imap = new Imap())
{
imap.ConnectSSL("imap.gmail.com");
imap.UseBestLogin("pat@gmail.com", "password");
// Select 'All Mail' folder
CommonFolders common = new CommonFolders(client.GetFolders());
client.Select(common.AllMail);
List<long> uids = imap.Search().Where(
Expression.GmailRawSearch(
"in:inbox subject:Notification with -without"));
foreach (MessageInfo info in imap.GetMessageInfoByUID(uids))
{
Console.WriteLine("{0} - {1}",
info.Envelope.GmailThreadId,
info.Envelope.Subject);
}
imap.Close();
}
' VB.NET version
Using imap As New Imap()
imap.ConnectSSL("imap.gmail.com")
imap.UseBestLogin("pat@gmail.com", "password")
' Select 'All Mail' folder
Dim common As New CommonFolders(client.GetFolders())
client.Select(common.AllMail)
Dim uids As List(Of Long) = imap.Search().Where( _
Expression.GmailRawSearch( _
"in:inbox subject:Notification with -without"))
For Each info As MessageInfo In imap.GetMessageInfoByUID(uids)
Console.WriteLine("{0} - {1}", _
info.Envelope.GmailThreadId, _
info.Envelope.Subject)
Next
imap.Close()
End Using
You can learn more about this Gmail IMAP extension here:
http://code.google.com/apis/gmail/imap/#x-gm-raw
Posted in Mail.dll | Tags: C#, Gmail, IMAP, IMAP component, VB.NET, X-GM-RAW